Homework 1
(10 points)
Tetris - Part 1
Overview
You will be implementing the game Tetris. Tetris is a deceptively simple, and
addictive puzzle game. Small pieces fall from the top of the grid to the bottom.
The pieces are comprised of 4 squares arranged into 7 different patterns. Players
must rotate the pieces as they fall and fit them together to complete lines. When
the player fills an entire line with blocks, that line is removed from the screen.
If the player cannot complete lines, the blocks will eventually fill to the top
of the screen and the game ends.
Concepts
The purpose of this assignment is to gain experience with the
following concepts:
- class relationships
- understanding and modifying provided code
- 2-dimensional arrays
- unit testing
Program Synopsis
In the real game of Tetris, a tetris piece, composed of
4 contiguous squares, falls from the top of the grid. The piece can be moved
left, right, or down as well as rotate clockwise. When the piece lands on the
bottom of the grid or another piece it becomes frozen, that is to say, a part of
the grid, and then another piece is created. If an entire row fills up with
parts of different pieces, then the entire row is removed and the rows above it
are moved down. Play continues until the pieces pile up to the top and a new
piece is created on top of already frozen pieces.
The starter code for this project implements a simple game that only
creates a single L-shaped piece that can move downwards. It uses elementary
keyboard input and graphics classes. For Homework #1, you will add
specific features to make it a more complete Tetris game. (Homework #2 will ask
you to produce a complete Tetris game that uses different shapes.)
Download the starter code: EventController.java,
Game.java, Grid.java,
LShape.java, Square.java,
Tetris.java, Direction.java
Here is a description of the different classes. These first 2 classes
have to do with the user interface
- Tetris - class to handle the graphics display. Also contains
the main method to start the game. You do not have to modify this class
at all. There is a lot of graphics code in here that you probably are
not familiar with. Don't worry; we will learn about it as the quarter
progresses.
- EventController - class to react to events (like key presses and
timer events) and tells the game what to do. You will not add any
methods to this class, but will have to modify an existing method. There
is alot of event handling code in here that you probably are not familiar
with. Don't worry; we will learn about it as the quarter progresses.
The rest of the classes have to do with modelling the game.
Except for the draw(Graphics g) methods, you should be able to read and
understand the rest of the code.
- Game - code that keeps track of the current piece and the
board. It contains the public methods to actually "play" the game.
- Grid - the grid is actually the board area on the screen where the
piece can move. Each square of the grid is represented as an element of a 2D array of Square objects. The upper left Square is at (0,0). The lower right Square is at (HEIGHT -1, WIDTH -1) where HEIGHT and WIDTH are the numbers of rows and columns in the grid. .The grid also keeps track of where previous pieces have
"frozen."
- LShape - the game piece
made of 4 squares stored in a 1D array of Square objects as shown in the picture.
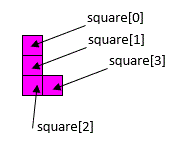
- Square - the building block for the LShape and the Grid
- Direction - an enum (short for enumeration) that lists all possible directions in the game of Tetris. An enum is a convenient way to group constants that are related. It also ensures that all of the variables of type Direction will have a value equal to one of the constants. More information about enums is given here.
Read through and become familiar with the sample code
provided. Determine what the different relations are between
different classes and what the different methods do. Drawings would come
in handy here.
Overview: For this homework, you will modify the game so that it
will produce another L-shaped piece once the current one has stopped moving.
In addition, you will add the ability for the L-shaped piece to move LEFT
and RIGHT . Finally, you will implement removal of solid rows from
the Grid.
Homework 2 will add the rest of the Tetris game features.
Program Details
- In order to get another piece to appear on the screen, the following line
of code needs to be added:
piece = new LShape(1, Grid.WIDTH/2 -1, grid);
Read through the starter code and find the correct place to put this line.
- Currently, the piece moves DOWN if the space key is pressed (this
will move it faster than it normally falls). Modify the game so that the piece
drops down if the space key is pressed. That is bring the moving piece on
top of the other pieces in just one step of the animation when the space bar
or the down arrow key are pressed.
The game piece also needs to be able to move LEFT and RIGHT if the corresponding
arrow keys are pressed. In order to handle these keys, you will
need to modify the method keyPressed(keyEvent e)
in the EventController class. You will find the correct constant names for
the associated keys in the KeyEvent class in the Java API.
In addition, you will have to modify some methods of one of the provided
classes in order to get the correct behavior. Reading and
understanding the code will make it clear which class you need to change.
- ROW-REMOVAL: Implement the method checkRows() in the Grid class.
This method should look through the grid and determine if there is a completely
filled in row (a row of all non EMPTY Squares). If such a row is found, it
should be removed and all the rows above it should be moved down. All
such rows should be found and removed in one call to checkRows. You
may find it useful to define a couple of private methods to help. See figure.
(Note that at this point, you only have the L-shaped piece to work with.
The example below shows what can happen when the other pieces have been implemented.)
Notice here that the bottom 2 rows are filled. They are removed and the
rows above are moved down.
Unit testing
- To practice unit testing, write two unit test methods (all within the same class, e.g. TetrisTest) to check the
correctness of the some of the key features of the game, namely
- the removal of one or more rows within the grid.
- and one other feature, e.g.
- the motion of a square (does it move to the right location? does canMove
work correctly)
- the motion of an L-shaped piece (does canMove work correctly? starting
from the left, does it move all the way to the right with the right number
of moves, does the drop down feature work well?)
- You may modify some of the existing classes to add methods that return the values of some of the instance fields to check them in your tests.
Report (should be typed and turned in as a pdf file with the java files on the class website)
Write a short statement that either says that you are in full compliance
with the requirements of the assignment or lists the ways in which you are
not (things that don't work).
- On paper draw an object diagram showing the relationships (has_a) between
the objects involved in the simulation of the tetris game. Describe (in plain
English) what happens in terms of messages being sent (= methods called) between
the objects when the left arrow key is pressed.
- Explain how you implemented the "drop-down" feature when the space
key is pressed.
- Describe the unit tests that you designed.
Individual evaluation report (should be typed and turned individually on Canvas)
Evaluate this project:
- What did you learn from it?
- Was it worth the effort?
- Did you feel that all team members contributed equally to the project?
Your program has to be your own.